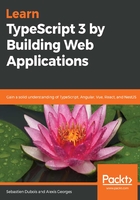
Listing existing todo items using lambda expressions
Speaking about the user experience, so far, we can add things to our todo list, but the only way to see the results is to open the web browser's console. We could certainly improve the user experience; let's give it a try!
In todo-it.ts, add a variable for the todoListContainer div element:
const todoListDiv: HTMLDivElement = document.getElementById('todoListContainer') as HTMLDivElement;
Add the following function:
function updateTodoList(): void { console.log("Updating the rendered todo list"); todoListDiv.innerHTML = ''; todoListDiv.textContent = ''; // Edge, ... const ul = document.createElement('ul'); ul.setAttribute('id', 'todoList'); todoListDiv.appendChild(ul); todoList.forEach(item => { const li = document.createElement('li'); li.setAttribute('class','todo-list-item'); li.innerText = item; ul.appendChild(li); }); }
This function first clears the content of the todoListContainer div. Then, it loops over the content of the todoList array, using the standard forEach function. Here is the reference for this function: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/forEach.
If you're familiar with other programming languages that support functional programming (FP) concepts, such as C#, Java, Python, Ruby, and so on, then you won't be surprised by the notation. Basically, what we pass to the forEach function is a function.
In ECMAScript 2015 and TypeScript jargon, people talk about arrow functions or fat-arrow functions. More generally, these are called lambda functions or lambda expressions. Let's call them lambdas for simplicity. In JavaScript and TypeScript, functions are first-class citizens—they can be treated like other types: passed as arguments to other functions and stored in variables.
The general syntax for lambdas in TypeScript is simple: (arguments) ⇒ { function body }.
By the way, take a look at the generated JavaScript code for the lambda: todoList.forEach(function (item) {...}).
TypeScript has converted our lambda expression to an anonymous function declaration; that way, our code is compatible with older environments, which is great. This is the beauty of TypeScript!
Let's continue.
Next, add the following code at the end of the if block (inside it) within the addTodo function, in order to update the todo list when new items are added:
// keep the list sorted todoList.sort(); // update the todo list updateTodoList();
Now give it a try. Add some tasks to your list, and you should see them appear on the screen (while remaining sorted). Yes, indeed, this is awesome!
Jokes apart, you can probably feel how painful it would be to maintain a complex user interface using only the DOM API. Others have felt that too, and that has led to the creation of libraries such as jQuery and, later, to the creation of frameworks such as Angular, React, and many others.