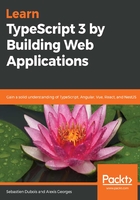
Adding todo items to the list
In order to allow the users to add items to the todo list, you need to add event handlers to the todoInput input and to the Add button.
Go back to index.html and replace the todoInput input with the following code:
<input type="text" id="todoInput" title="What should be added to the todo list?" onblur="addTodo()" />
Then, replace the button input with the following:
<input type="button" value="Add todo" onclick="addTodo()" />
In the case of todoInput, we have added an onblur event handler; it will be called whenever the user leaves the field. In the case of the button, we have added an onclick handler.
In both cases, we simply invoke the addTodo function. Let's implement that one now:
function addTodo(): void { // if we don't have the todo input if(todoInput == null) { console.error('The todo input is missing from the page!'); return; } // get the value from the input const newTodo: string = todoInput.value; // verify that there is text if ('' !== newTodo.trim()) { console.log('Adding todo: ', newTodo); // add the new item to the list todoList.push(newTodo); console.log('New todo list: ', todoList); // clear the input todoInput.value = ''; } }
Notice that our function's return type is void. This is how you tell TypeScript not to expect anything in return from the function. With that defined, TypeScript will complain if you do return a value. Defining the return type of your functions is very important, as that will help you avoid many bugs.
Did you notice how VS Code helps with autocompletion as seen in the following screenshot:

This is really where TypeScript shines.