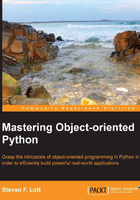
Containers and collections
The collections
module defines a number of collections above and beyond the built-in container classes. The container classes include namedtuple()
, deque
, ChainMap
, Counter
, OrderedDict
, and defaultdict
. All of these are examples of classes based on ABC definitions.
The following is a quick interaction to show how we can inspect collections to see the methods they will support:
>>> isinstance( {}, collections.abc.Mapping ) True >>> isinstance( collections.defaultdict(int), collections.abc.Mapping ) True
We can inspect the simple dict
class to see that it follows the basic mapping protocol and will support the required methods.
We can inspect a defaultdict
collection to confirm that it is also a mapping.
When creating a new kind of container, we can do it informally. We can create a class that has all of the right special methods. However, we aren't required to make a formal declaration that it's a certain kind of container.
It's more clear (and more reliable) to use a proper ABC as the base class for one of our application classes. The additional formality has the following two advantages:
- It advertises what our intention was to people reading (and possibly using or maintaining) our code. When we make a subclass of
collections.abc.Mapping
, we're making a very strong claim about how that class will be used. - It creates some diagnostic support. If we somehow fail to implement all of the required methods properly, we can't create instances of the abstract base class. If we can't run the unit tests because we can't create instances of an object, then this indicates a serious problem that needs to be fixed.
The entire family tree of built-in containers is reflected in the abstract base classes. Lower-level features include Container
, Iterable
, and Sized
. These are a part of higher-level constructs; they require a few specific methods, particularly __contains__()
, __iter__()
, and __len__()
, respectively.
Higher-level features include the following characteristics:
Sequence
andMutableSequence
: These are the abstractions of the concrete classeslist
andtuple
. Concrete sequence implementations also includebytes
andstr
.MutableMapping
: This is the abstraction ofdict
. It extendsMapping
, but there's no built-in concrete implementation of this.Set
andMutableSet
: These are the abstractions of the concrete classes,frozenset
andset
.
This allows us to build new classes or extend existing classes and maintain a clear and formal integration with the rest of Python's built-in features.
We'll look at containers and collections in detail in Chapter 6, Creating Containers and Collections.