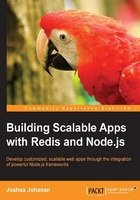
What this book covers
Chapter 1, Backend Development with Express, shows us how to serve our pages using Express. Express is a full-featured web application framework that provides us with many features while writing very little code. It also has a rich middleware system that others have extended. This middleware allows us to work with form data as well as use templates and sessions. We will build the foundation of the application, on which all the other chapters will be based.
Chapter 2, Extending Our Development with Socket.IO, shows us how to build real-time applications using WebSockets. WebSockets are the next step in the evolution of dynamic web pages that allow users to interact instantaneously. This chapter also covers the use of tying Socket.IO to the sessions that Express creates.
Chapter 3, Authenticating Users, shows us how to build a login page that actually works! We will be using the Passport framework to build our authentication functions. Passport has performed a lot of heavy lifting in building connectors to different providers. Many of these implemented OAuth or OAuth 2.0. If you have ever had to develop against these different OAuth providers, you can appreciate the work that went into each library. You will also be shown how to store local passwords securely.
Chapter 4, RabbitMQ for Message Queuing, covers the topic of message queues. These are a requirement of any scalable application, allowing you to break your application up, that serves both its complexity and scope. In this chapter, we will cover some great use cases for this. In addition, you will be able to build your own message queues and tie them to functions.
Chapter 5, Adopting Redis for Application Data, shows us how to use the store information and retrieve it from Redis. This is important, as the Redis data storage engine is unlike any relational database. Thinking of it as such can actually create issues! We will cover the commands you will use the most throughout your application, as well as take a look at how Redis implements message queuing in your application.
Chapter 6, Using Bower to Manage Our Frontend Dependencies, begins to take a look at how you can begin the frontend development of your application. We will not have an application without a frontend. We will talk about the frameworks that will be used and why they are chosen.
Chapter 7, Using Backbone and React for DOM Events, covers the backbone, if you can excuse the pun, of the frontend of our application. The two most important tasks when using JavaScript in a browser are DOM manipulation and responding to events. You will learn how to listen for real-time events and then interact with the page. Backbone and React will help us build the maintainable code to do this.
Chapter 8, JavaScript Best Practices for Application Development, shows us how to build better JavaScript. JavaScript, as a scripting language, will run despite making many mistakes, which is both a good and a bad thing. However, you will still need to know if you have forgotten a semicolon or caused a runtime error. We will achieve this by building a repeatable build system. You will also be shown modules and how to module proof the code.
Chapter 9, Deployment and Scalability, shows us how to remove our site off localhost. It is critical to get a deployment script right, as it is very easy to miss a step when deploying. We will cover how to deploy to one, two, or more servers, including having different environments from which we can deploy. The advantage of these multiple servers for your application is that it is horizontally scalable, making it easy to add more servers.
Chapter 10, Debugging and Troubleshooting, shows us how to look at the context of a function call because strewing console.log() functions everywhere is a horrible method to debug. We will also learn how to track memory leaks in both the frontend and backend. If you have had experience in debugging JavaScript in Chrome, you will feel right at home here.