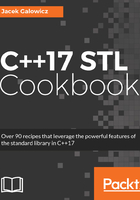
How it works...
In C++17, std::map got a new member function extract. It comes in two flavors:
node_type extract(const_iterator position);
node_type extract(const key_type& x);
In this recipe, we used the second one, which accepts a key and then finds and extracts the map node that matches the key parameter. The first one accepts an iterator, which implies that it is faster because it doesn't need to search for the item.
If we try to extract an item that doesn't exist with the second method (the one that searches using a key), it returns an empty node_type instance. The empty() member method returns us a Boolean value that tells whether a node_type instance is empty or not. Accessing any other method on an empty instance leads to undefined behavior.
After extracting nodes, we were able to modify their keys using the key() method, which gives us nonconst access to the key, although keys are usually const.
Note that in order to reinsert the nodes into the map again, we had to move them into the insert function. This makes sense because extract is all about avoiding unnecessary copies and allocations. Note that while we move a node_type instance, this does not result in actual moves of any of the container values.