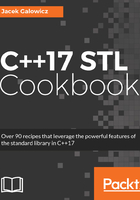
上QQ阅读APP看书,第一时间看更新
How to do it...
In this section, we will insert multiple items into an std::map, and use insertion hints for that, in order to reduce the number of lookups.
- We are mapping strings to numbers, so we need the header files included for std::map and std::string.
#include <iostream>
#include <map>
#include <string>
- The next step is to instantiate a map, which already contains some example characters.
int main()
{
std::map<std::string, size_t> m {{"b", 1}, {"c", 2}, {"d", 3}};
- We will insert multiple items now, and for each item we will use an insertion hint. Since we have no hint in the beginning to start with, we will just do the first insertion pointing to the end iterator of the map.
auto insert_it (std::end(m));
- We will now insert items from the alphabet backward while always using the iterator hint we have, and then reinitialize it to the return value of the insert function. The next item will be inserted just before the hint.
for (const auto &s : {"z", "y", "x", "w"}) {
insert_it = m.insert(insert_it, {s, 1});
}
- And just for the sake of showing how it is not done, we insert a string which will be put at the leftmost position in the map, but give it a completely wrong hint, which points to the rightmost position in the map--the end.
m.insert(std::end(m), {"a", 1});
- Finally, we just print what we have.
for (const auto & [key, value] : m) {
std::cout << "\"" << key << "\": " << value << ", ";
}
std::cout << '\n';
}
- And this is the output we get when we compile and run the program. Obviously, the wrong insertion hint did not hurt too much, as the map ordering is still correct.
"a": 1, "b": 1, "c": 2, "d": 3, "w": 1, "x": 1, "y": 1, "z": 1,