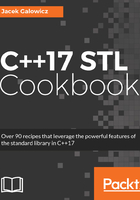
上QQ阅读APP看书,第一时间看更新
How to do it...
In this recipe, we use the initializer syntax in both the supported contexts in order to see how they tidy up our code:
- The if statements: Imagine we want to find a character in a character map using the find method of std::map:
if (auto itr (character_map.find(c)); itr != character_map.end()) {
// *itr is valid. Do something with it.
} else {
// itr is the end-iterator. Don't dereference.
}
// itr is not available here at all
- The switch statements: This is how it would look to get a character from the input and, at the same time, check the value in a switch statement in order to control a computer game:
switch (char c (getchar()); c) {
case 'a': move_left(); break;
case 's': move_back(); break;
case 'w': move_fwd(); break;
case 'd': move_right(); break;
case 'q': quit_game(); break;
case '0'...'9': select_tool('0' - c); break;
default:
std::cout << "invalid input: " << c << '\n';
}