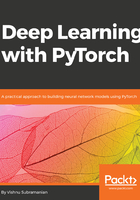
The PyTorch way of building deep learning algorithms
All the networks in PyTorch are implemented as classes, subclassing a PyTorch class called nn.Module, and should implement __init__ and forward methods. Inside the init function, we initialize any layers, such as the linear layer, which we covered in the previous section. In the forward method, we pass our input data into the layers that we initialized in our init method and return our final output. The non-linear functions are often directly used in the forward function and some use it in the init method too. The following code snippet shows how a deep learning architecture is implemented in PyTorch:
class MyFirstNetwork(nn.Module):
def __init__(self,input_size,hidden_size,output_size):
super(MyFirstNetwork,self).__init__()
self.layer1 = nn.Linear(input_size,hidden_size)
self.layer2 = nn.Linear(hidden_size,output_size)
def __forward__(self,input):
out = self.layer1(input)
out = nn.ReLU(out)
out = self.layer2(out)
return out
If you are new to Python, some of the preceding code could be difficult to understand, but all it is doing is inheriting a parent class and implementing two methods in it. In Python, we subclass by passing the parent class as an argument to the class name. The init method acts as a constructor in Python and super is used to pass on arguments of the child class to the parent class, which in our case is nn.Module.