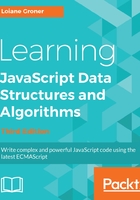
References for JavaScript array methods
Arrays in JavaScript are modified objects, meaning that every array we create has a few methods available to be used. JavaScript arrays are very interesting because they are very powerful and have more capabilities available than primitive arrays in other languages. This means that we do not need to write basic capabilities ourselves, such as adding and removing elements in/from the middle of the data structure.
The following is a list of the core available methods in an array object. We have covered some methods already:
Method |
Description |
concat |
Joins multiple arrays and returns a copy of the joined arrays. |
every |
Iterates every element of the array, verifying the desired condition (function) until false is returned. |
filter |
Creates an array with each element that evaluates to true in the function provided. |
forEach |
Executes a specific function on each element of the array. |
join |
Joins all the array elements into a string. |
indexOf |
Searches the array for specific elements and returns its position. |
lastIndexOf |
Returns the position of the last item in the array that matches the search criterion. |
map |
Creates a new array from a function that contains the criterion/condition and returns the elements of the array that match the criterion. |
reverse |
Reverses the array so that the last item becomes the first and vice versa. |
slice |
Returns a new array from the specified index. |
some |
Iterates every element of the array, verifying the desired condition (function) until true is returned. |
sort |
Sorts the array alphabetically or by the supplied function. |
toString |
Returns the array as a string. |
valueOf |
Similar to the toString method, returns the array as a string. |
We have already covered the push, pop, shift, unshift, and splice methods. Let's take a look at these new ones. These methods will be very useful in the subsequent chapters of this book, where we will code our own data structure and algorithms. Some of these methods are very useful when we work with functional programming, which we will cover in Chapter 14, Algorithm Designs and Techniques.