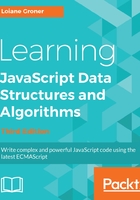
上QQ阅读APP看书,第一时间看更新
Default parameter values for functions
With ES2015, it is also possible to define default parameter values for functions. The following is an example:
function sum(x = 1, y = 2, z = 3) { return x + y + z; } console.log(sum(4, 2)); // outputs 9
As we are not passing z as a parameter, it will have a value of 3 by default. So, 4 + 2 + 3 == 9.
Before ES2015, we would have to write the preceding function as in the following code:
function sum(x, y, z) { if (x === undefined) x = 1; if (y === undefined) y = 2; if (z === undefined) z = 3; return x + y + z; }
Or, we could also write the code as follows:
function sum() { var x = arguments.length > 0 && arguments[0] !== undefined ? arguments[0] : 1; var y = arguments.length > 1 && arguments[1] !== undefined ? arguments[1] : 2; var z = arguments.length > 2 && arguments[2] !== undefined ? arguments[2] : 3; return x + y + z; }
JavaScript functions also have a built-in object called the arguments object. The arguments object is an array of the arguments used when the function is called. We can dynamically access and use the arguments even if we do not know the argument name.
With ES2015, we can save a few lines of code using the default parameter values functionality.
The preceding example can be executed at https://goo.gl/AP5EYb.