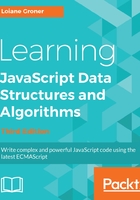
Variables
Variables store data that can be set, updated, and retrieved whenever necessary. Values that are assigned to a variable belong to a type. In JavaScript, the available types are number, string, boolean, function, and object. We also have undefined and null, along with arrays, dates, and regular expressions.
Although JavaScript has different available variable types, it is not a strongly typed language such as C/C++, C#, and Java. In strongly typed languages, we need to declare the type of the variable along with its declaration (for example, in Java, to declare an integer variable, we use int num = 1;). In JavaScript, we only need to use the keyword var, and we do not need to declare the variable type. For this reason, JavaScript is not a strongly typed language. However, there are discussions and a specification in draft mode for optional static typing (https://github.com/dslomov/typed-objects-es7) that can become part of the JavaScript specification (ECMAScript) in the future. We can also use TypeScript in case we want to type our variables when working with JavaScript. We will learn more about ECMAScript and TypeScript later in this chapter.
The following is an example of how to use variables in JavaScript:
var num = 1; // {1} num = 3; // {2} var price = 1.5; // {3} var myName = 'Packt'; // {4} var trueValue = true; // {5} var nullVar = null; // {6} var und; // {7}
- In line {1}, we have an example of how to declare a variable in JavaScript (we are declaring a number). Although it is not necessary to use the var keyword declaration, it is a good practice to always specify when we declare a new variable.
- In line {2}, we updated an existing variable. JavaScript is not a strongly typed language. This means you can declare a variable, initialize it with a number, and then update it with a string or any other datatype. Assigning a value to a variable that is different from its original type is also not a good practice.
- In line {3}, we also declared a number, but this time it is a decimal floating point. In line {4}, we declared a string; in line {5}, we declared a boolean. In line {6}, we declared a null value, and in line {7}, we declared an undefined variable. A null value means no value, and undefined means a variable that has been declared but not yet assigned a value.
If we want to see the value of each variable we declared, we can use console.log to do so, as listed in the following code snippet:
console.log('num: ' + num); console.log('myName: ' + myName); console.log('trueValue: ' + trueValue); console.log('price: ' + price); console.log('nullVar: ' + nullVar); console.log('und: ' + und);
The console.log method also accepts more than just arguments. Instead of console.log('num: ' + num), we can also use console.log('num: ', num). While the first option is going to concatenate the result into a single string, the second option allows us to add a description and also visualize the variable content in case it is an object.
We will discuss functions and objects later in this chapter.