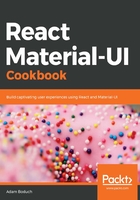
上QQ阅读APP看书,第一时间看更新
How to do it...
Let's say that you have a component that renders drawer navigation using the Drawer component. Instead of writing the items state directly in the component markup, you want to have the items state stored in the state of the component. For example, in response to permission checks on the user, items might be disabled or completely hidden.
Here's an example that uses an array of item objects from the component state:
import React, { useState } from 'react';
import Drawer from '@material-ui/core/Drawer';
import Grid from '@material-ui/core/Grid';
import Button from '@material-ui/core/Button';
import List from '@material-ui/core/List';
import ListItem from '@material-ui/core/ListItem';
import ListItemIcon from '@material-ui/core/ListItemIcon';
import ListItemText from '@material-ui/core/ListItemText';
import Typography from '@material-ui/core/Typography';
import HomeIcon from '@material-ui/icons/Home';
import WebIcon from '@material-ui/icons/Web';
export default function DrawerItemState() {
const [open, setOpen] = useState(false);
const [content, setContent] = useState('Home');
const [items] = useState([
{ label: 'Home', Icon: HomeIcon },
{ label: 'Page 2', Icon: WebIcon },
{ label: 'Page 3', Icon: WebIcon, disabled: true },
{ label: 'Page 4', Icon: WebIcon },
{ label: 'Page 5', Icon: WebIcon, hidden: true }
]);
const onClick = content => () => {
setOpen(false);
setContent(content);
};
return (
<Grid container justify="space-between">
<Grid item>
<Typography>{content}</Typography>
</Grid>
<Grid item>
<Drawer open={open} onClose={() => setOpen(false)}>
<List>
{items
.filter(({ hidden }) => !hidden)
.map(({ label, disabled, Icon }, i) => (
<ListItem
button
key={i}
disabled={disabled}
onClick={onClick(label)}
>
<ListItemIcon>
<Icon />
</ListItemIcon>
<ListItemText>{label}</ListItemText>
</ListItem>
))}
</List>
</Drawer>
</Grid>
<Grid item>
<Button onClick={() => setOpen(!open)}>
{open ? 'Hide' : 'Show'} Drawer
</Button>
</Grid>
</Grid>
);
}
This is what the drawer looks like when you click on the SHOW DRAWER button:

If you select one of these items, the drawer will close and the content of the screen will be updated; for example, after clicking on Page 2, you should see something similar to the following screenshot:
