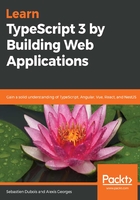
Defining and executing npm scripts
Scripts are an awesome feature of npm that we will use throughout the book and that we heavily recommend not overlooking.
Scripts are sets of commands that you can include in the package.json file of your projects in order to automate various tasks. An example of a task that you could automate using scripts is the compilation of your project (for example, in our case, the transpilation from TypeScript to JavaScript).
Using scripts, you can define fairly complex build systems for your applications. If you want a real-world example, check this package.json file out: https://github.com/NationalBankBelgium/stark/blob/master/package.json.
With a newly initialized npm project, you usually only have the following:
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
Inside the scripts block, you can actually define as many scripts as you'd like. Each entry has a name (the one you'll use to execute the script) and a body containing the command(s) to execute when invoking the script.
In those scripts, you can use any command available in your current path (for example, bash, npm, ls, mkdir, and many others), as well as any binary located under node_modules/.bin.
Here's an example to invoke karma as part of the test script:
"scripts": {
"test": "karma"
},
To invoke a script, you simply have to execute npm run <script name>. You can try it out with the previous example.
For some special scripts such as test, you may also use shorthand: npm test. Here's an example of the output:
$ npm test > npm-install-example@1.0.0 test C:\dev\wks\tsbook\assets\2\npm-install-example > karma Command not specified. Karma - Spectacular Test Runner for JavaScript. ... Commands: start [<configFile>] [<options>] Start the server / do single run. init [<configFile>] Initialize a config file. run [<options>] [ -- <clientArgs>] Trigger a test run. completion Shell completion for karma. Run --help with particular command to see its description and available options. Options: --help Print usage and options. --version Print current version. npm ERR! Test failed. See above for more details.
We'll look at more examples throughout the book.
Check out the official documentation if you want to know more about npm scripts: https://docs.npmjs.com/misc/scripts.