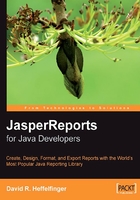
Generating the Report
In JasperReports lingo, the process of generating a report from a report template, or Jasper file, is known as filling the report. Reports are filled programmatically, by calling the fillReportToFile()
method in the net.sf.jasperreports.engine.JasperFillManager
class. The fillReportToFile()
method fills a report and saves it to disk.
There are six overloaded versions of the fillReportToFile()
method, which are listed below:
JasperFillManager.fillReportToFile(JasperReport jasperReport, String destFileName, Map parameters, Connection connection)
JasperFillManager.fillReportToFile(JasperReport jasperReport, String destFileName, Map parameters, JRDataSource datasource)
JasperFillManager.fillReportToFile(String sourceFileName, Map parameters, Connection connection)
JasperFillManager.fillReportToFile(String sourceFileName, Map parameters, JRDatasource dataSource)
JasperFillManager.fillReportToFile(String sourceFileName, String destFileName, Map parameters, Connection connection)
JasperFillManager.fillReportToFile(String sourceFileName, String destFileName, Map parameters, JRDataSource dataSource)
The following table illustrates the parameters used in these methods:

As can be seen above, in most cases, we pass data for filling reports via an instance of a class implementing the net.sf.jasperreports.engine.JRDataSource
interface. Report templates can have embedded SQL queries. These SQL queries are defined inside the<queryString>
element in the JRXML file. For reports that contain an SQL query, instead of passing a JRDataSource
, we pass an instance of a class implementing the java.sql.Connection
interface. JasperReports then uses this Connection
object to execute the query and obtain the report data from the database.
Our report contains only static text; there is no dynamic data displayed in the report. There is no way to fill a report without passing either a JRDataSource
or a Connection
. JasperReports provides an implementation of the JRDataSource
containing no data. The class is named, appropriately enough, JREmptyDataSource
. Since our report takes no parameters, passing an empty instance of java.util.HashMap
will be enough for our purposes. We will follow the recommended approach of naming our report using the same name as the one used for the report template (except for the extension). Given all of these facts, the most appropriate version of fillReportToFile()
for our report is the fourth version. Here is its signature again:
JasperFillManager.fillReportToFile(String sourceFileName, Map parameters, JRDataSource dataSource)
The following Java class fills the report and saves it to disk:
package net.ensode.jasperbook;
import java.util.HashMap;
import net.sf.jasperreports.engine.JREmptyDataSource;
import net.sf.jasperreports.engine.JRException;
import net.sf.jasperreports.engine.JasperFillManager;
public class FirstReportFill
{
public static void main(String[] args)
{
try
{
System.out.println("Filling report...");
JasperFillManager.fillReportToFile("reports/FirstReport.jasper", new HashMap(), new JREmptyDataSource());
System.out.println("Done!");
}
catch (JRException e)
{
e.printStackTrace();
}
}
}
After executing the above class, we should have a file named FirstReport.JRprint
in the same location as our compiled report template, which was named FirstReport.jasper
.
Viewing the Report
JasperReports includes a utility class, net.sf.jasperreports.view.JasperViewer
, that we can use to view generated reports. As with the tool to preview designs, the easiest way to use it is to wrap it into an ANT target. Again, this is the approach taken by the samples included with JasperReports, and the approach taken by us as well. Let us add a new target to our ANT build file. Following the conventions established by the JasperReports samples, we will name this target view
.
<project name="FirstReport XML Design Preview" default="viewDesignXML" basedir=".">
<description>Previews and compiles our First Report</description>
<property name="file.name" value="FirstReport"/>
<!-- Directory where the JasperReports project file was extracted,
needs to be changed to match the local environment -->
<property name="jasper.dir" value="/usr/local/share/java/jasperreports-1.1.0"/>
<property name="classes.dir" value="${jasper.dir}/build/classes"/>
<property name="lib.dir" value="${jasper.dir}/lib"/>
<path id="classpath">
<pathelement location="./"/>
<pathelement location="${classes.dir}"/>
<fileset dir="${lib.dir}">
<include name="**/*.jar"/>
</fileset>
</path>
<target name="viewDesignXML"
description="Launches the design viewer to preview the XML report design.">
<java classname="net.sf.jasperreports.view.JasperDesignViewer" fork="true">
<arg value="-XML"/>
<arg value="-F${file.name}.jrxml"/>
<classpath refid="classpath"/>
</java>
</target>
<target name="viewDesign"
description="Launches the design viewer to preview the compiled report design.">
<java classname="net.sf.jasperreports.view.JasperDesignViewer" fork="true">
<arg value="-F${file.name}.jasper"/>
<classpath refid="classpath"/>
</java>
</target>
<target name="compile"
description="Compiles the XML report design and produces the .jasper file.">
<taskdef name="jrc" classname="net.sf.jasperreports.ant.JRAntCompileTask">
<classpath refid="classpath"/>
</taskdef>
<jrc destdir=".">
<src>
<fileset dir=".">
<include name="**/*.jrxml"/>
</fileset>
</src>
<classpath refid="classpath"/>
<jrc>
</target>
<target name="view" description="Launches the report viewer to preview the report stored in the .JRprint file."> <java classname="net.sf.jasperreports.view.JasperViewer" fork="true"> <arg value="-F${file.name}.JRprint"/> <classpath refid="classpath"/> </java> </target>
</project>
After executing the new view
ANT target, we should see a window like the following:

That's it! We have successfully created our first report.